Azure AD app do not honor conditional access policies levaraging IP restrictions.
Suppose we have a conditional access policy which restricts access to any app from any IP except certain IP ranges via a named location (in this case using my ISP network).
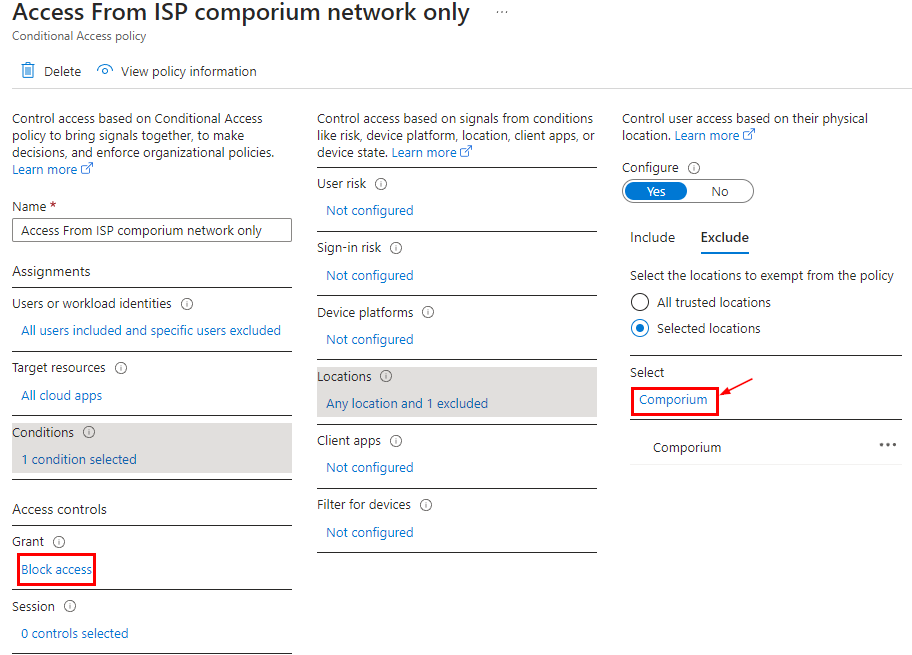
Interactive user login – Blocked
You will notice the user interactive sign-in gets blocked when coming from an IP outside of what is allowed.
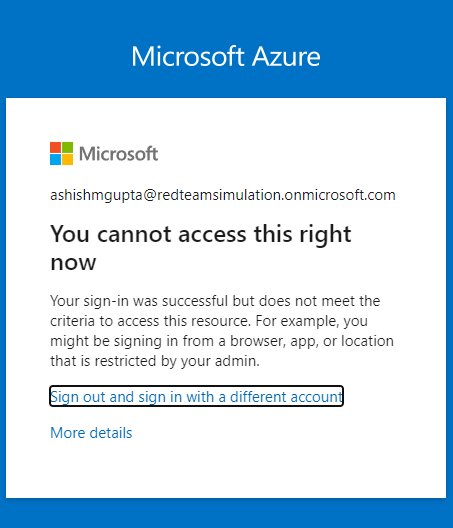

Login using a service principal for Azure AD app – Allowed
In this section, we will see if you have credentials for Azure AD app, you can access resources depending on what permissions the app has. In this example, we would read all the emails.
Setup
Lets setup an Azure AD app with mail.read permission and a credential.
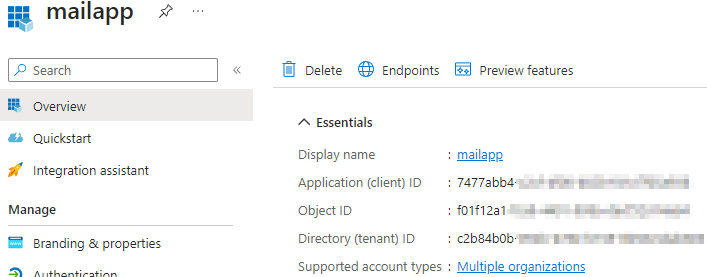
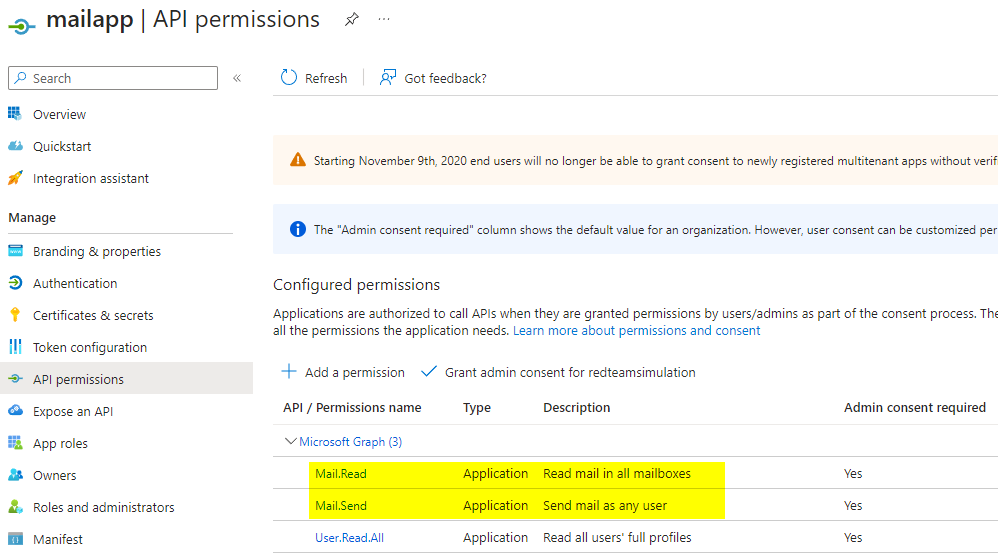
Code to get emails from all the mailboxes
Prerequisites : Install and import ExchangeOnlinemanagement and Microsoft.Graph modules
Install-Module ExchangeOnlineManagement
Import-Module ExchangeOnlineManagement
Install-Module Microsoft.Graph
Import-Module Microsoft.Graph
Replace the clientId and tenantId with the clientId of the app and the tenant id for your tenant respectively. When the script is run, please supply the credential created for the app.
# Import the required module
Import-Module Microsoft.Graph
$err_string= ''
# Set the necessary variables
$clientId = "7477abb4-xxxx-xxxx-xxxx-xxxxxx"
$tenantId = "c2b84b0b-xxxx-xxxx-xxxx-xxxxxxx"
$ClientSecretCredential = Get-Credential -Credential $clientId
# Connect to Microsoft Graph
Connect-MgGraph -TenantId $tenantId -ClientSecretCredential $ClientSecretCredential -NoWelcome
# Get all users in the tenant
$users = Get-MgUser
# Loop through each user
foreach ($user in $users) {
# Get the user's mailbox
try {
$mailbox = Get-MgUserMailFolderMessage -UserId $user.Id -MailFolderId 'Inbox' -ErrorAction Stop
$test = $user.Mail
write-host "####### Reading emails for mailbox " -nonewline
write-host $test -foreground red -nonewline
write-host " ##########"
write-host "Found " -nonewline
write-host $mailbox.Length -foreground red -nonewline
write-host " email(s) "
foreach ($message in $mailbox) {
# Print the message subject and received date
Write-Output (" ----------------------------------------------------")
Write-Output ("Subject: " + $message.Subject)
Write-Output ("Received: " + $message.ReceivedDateTime)
$body = $message.Body.Content -replace '<[^>]+>',''
$body = $body.trim()
Write-Output ("Body: " + $body)
}
write-host "`n"
}
catch
{
$err_string = $_ | Out-String
}
if ($err_string -inotmatch "The mailbox is either inactive, soft-deleted, or is hosted on-premise")
{
Write-Host $err_string
}
}
# Disconnect from Microsoft Graph
Disconnect-MgGraph
Running the above code by supplying the secret for the below and we can see we are still able to access all the emails. The service principle sign-in logs clearly note the access is from outside the IP address (from a foreign country) but the conditional access policy didn’t apply.
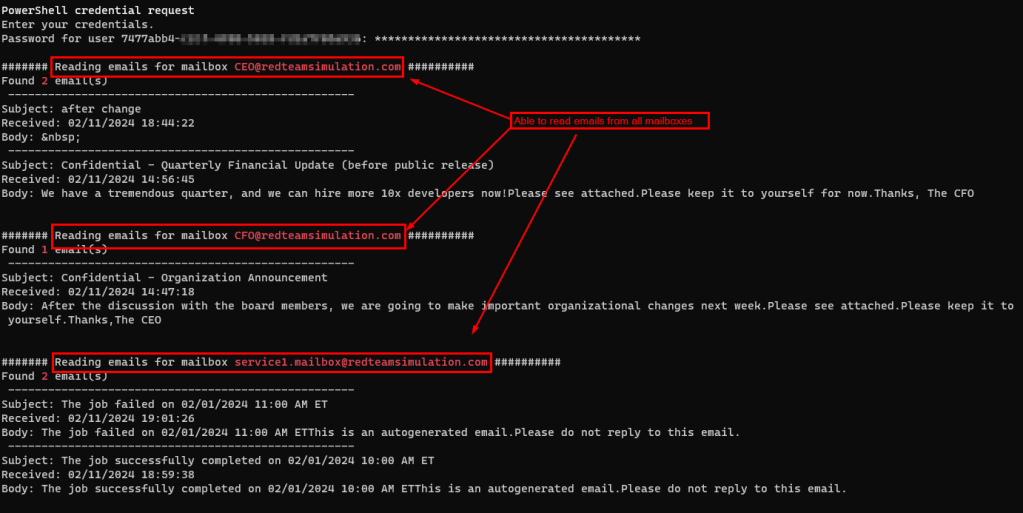

Using Microsoft Entra Workload ID to implement the conditional access
To address this, Microsoft has a new feature named Microsoft Entra Workload ID. Bad thing here is It needs Its own premium license. Good thing is you can try it out to see if this even works!
Login to the Entra ID portal as a global admininstrator and search for Workload Identities and activate the trial of 200 licenses for 90 days.
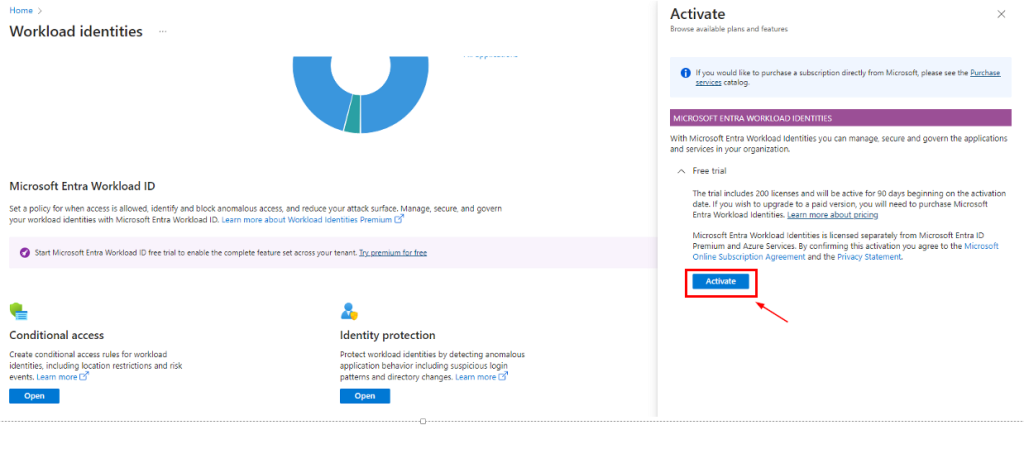
Then login to Microsoft Admin portal, and assign the users with “Micsoroft Entra Workload ID” license.
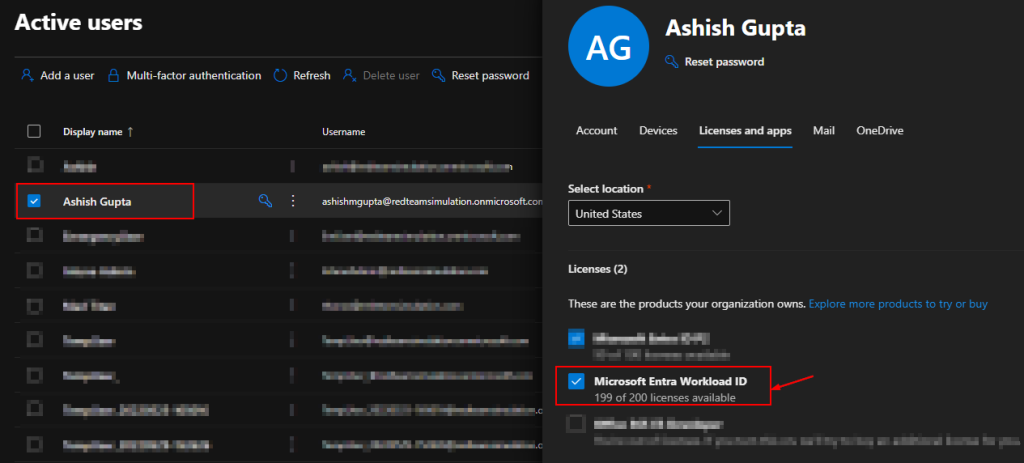
Once the license is assigned, login as the GA and licensed user to the the Entra portal.
Go to Protection > Conditional Access > Create.
There we see “Workload Identities” under “What does this policy apply to”.
Now, we can select the app we want to apply the conditional access with IP restriction.
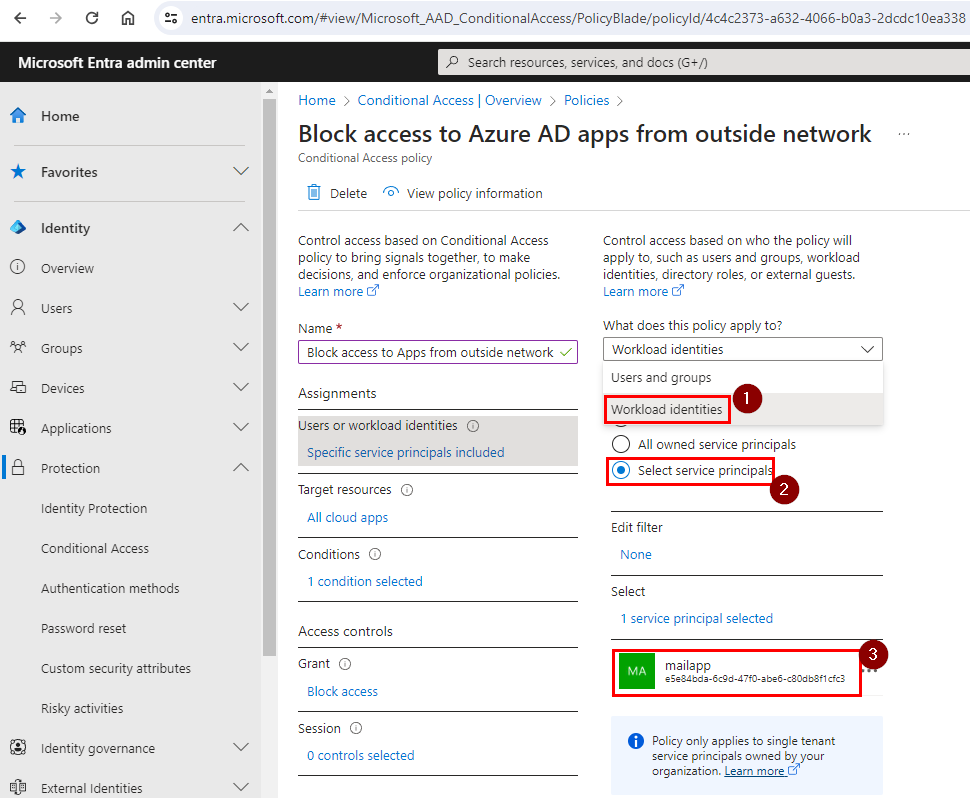
Running the same code above would now show error noting the access has been blocked by the conditional access policy
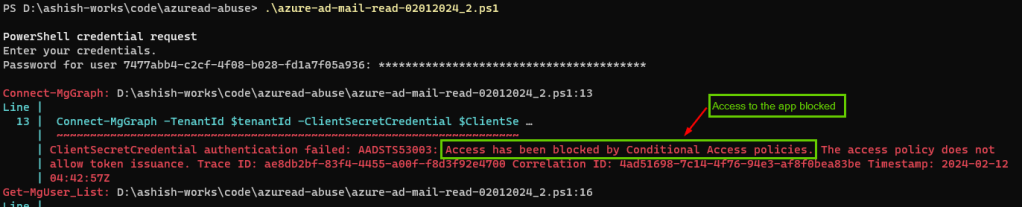
Service principal sign-in logs would show the failure as well

Conclusion
Microsoft Entra Workload ID premium looks promising and goes much beyond the conditional access. Its worth looking at its capabilities.
https://www.microsoft.com/en-us/security/business/identity-access/microsoft-entra-workload-id